Discussion Session
Topic:
C++ in a UNIX Environment
Advanced Session
Lead - Harsha
Starter code
Problem:
Given a node that represents the head of a linked list, find where there exists a circular loop. Note: The linked list need not necessarily wrap to back to the head node.
Solution: O(max(N,M))
Problem:
Given two nodes that represent that heads of two different linked lists, identify whether the linked lists are connected at any point.
Solution: O(max(M,N))
Advanced Session
Lead - Harsha
Problem:
Given a two dimensional ArrayList of boolean values that represent a maze where false represents a wall and true represents an open space, write a function that returns whether a path exists from the start to the end. Assume index (0,0) is the start of the maze and (n,n) represents the end. Assume that there only exists one path from start to end. Use the following code to initialize your arrayList. Diagonals do not count as a path.
Starter code for ArrayList
Solution: Recursive O(MN)
Advanced Session
Lead - Atharv
Activity: The Pie of Life
- Draw one large circle on a piece of paper to represent a typical day in your life
- Divide the circle into different slices
- Each slice represents the amount of time you spend doing different activities
- Ex: Sleeping, playing an instrument, studying, hanging with friends, physical activity
- Share and guess which pie belongs to whom
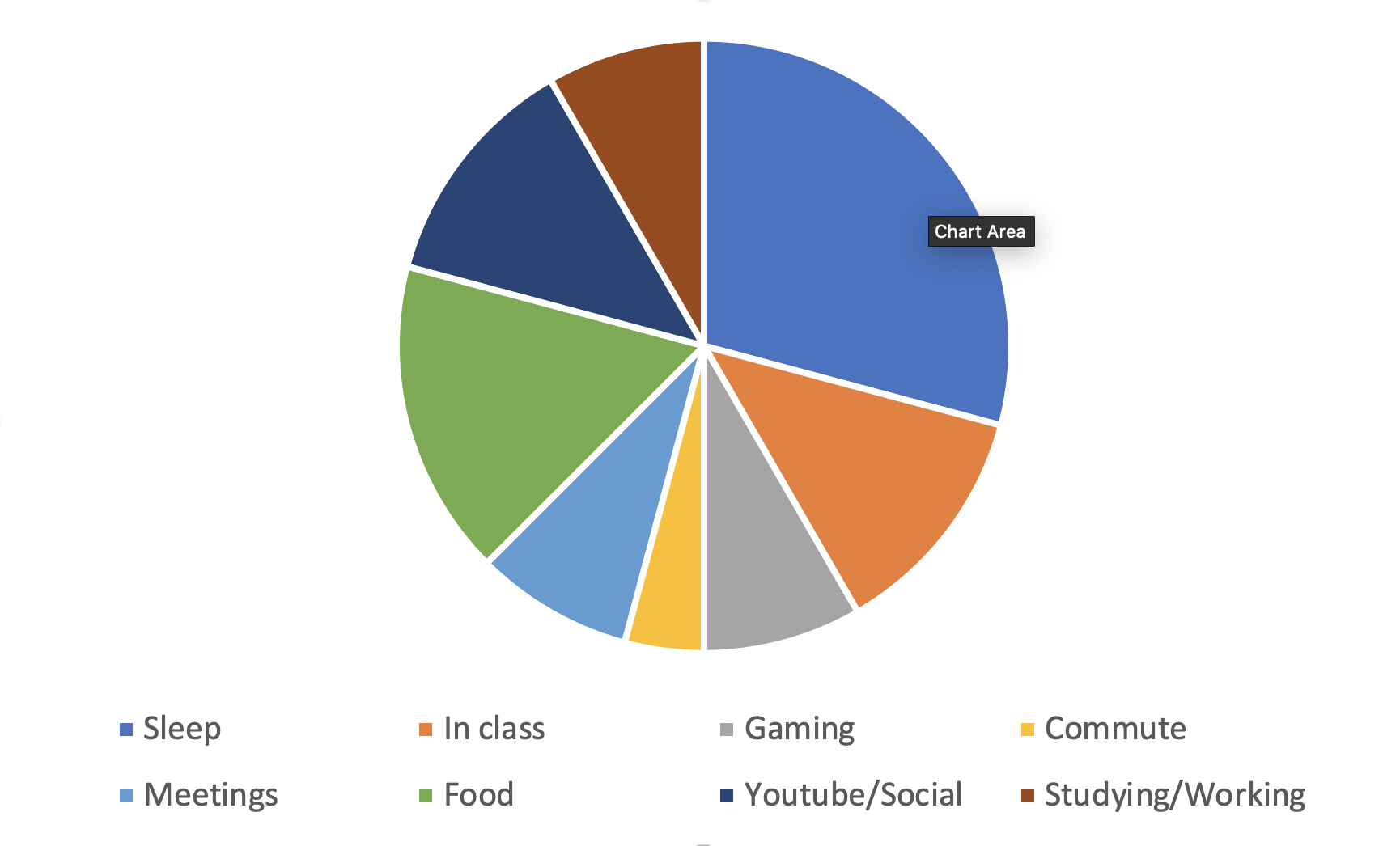
Problem:
Given n non-negative integers a1, a2, ..., an, where each represents a point at coordinate (i, ai). n vertical lines are drawn such that the two endpoints of line i is at (i, ai) and (i, 0). Find two lines, which together with x-axis forms a container, such that the container contains the most water.
Note: You may not slant the container and n is at least 2.
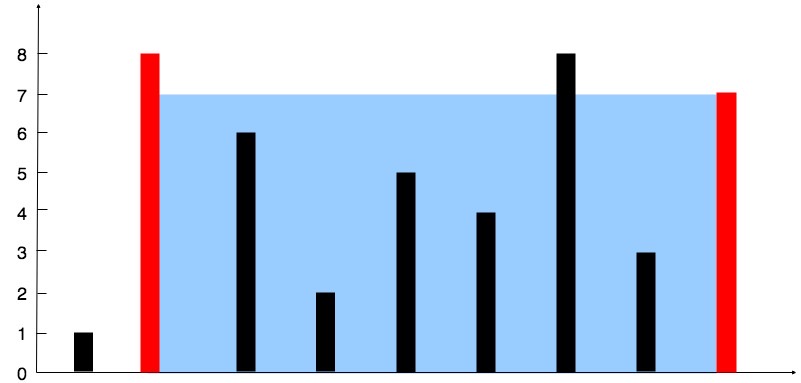
Solution: O(n2)
Solution: O(N)
Novice Session
Leads - Ifrit & Harsha
Activity: Who Knows Who Best?
Split in 2 teams. Every person on the other team has 30 seconds to write down their answer on a piece of paper. Crumble the paper up. The other team tries to guess who wrote what answer down as a team by writing down their guesses on the whiteboard. After guesses are made for the round the answers are revealed. At the end of every round the points are tallied up. After 5 rounds, whichever team has the most amount of points win.
Tutorial: Time & Space Complexity
Problem:
Given a list of Integers with n elements and a target, find the set of factors that multiply to the target. Challenge: And if none exist then provide the closest.
public static void findMultiple(List< Integer> list, int target) {
}
Solution: O(N2)
Solution: O(N)
Advanced Session
✭ Lead - Sanjeev ✭
Activity: Turning Over A New Leaf
Based on the number of people in this activity, make sure you use a cloth big enough for everyone to fit one. The challenge in this game is that with everyone standing on the piece of cloth must work together to flip the piece of cloth completely. There are only 2 rules that you must obey! One is no one can step outside the piece of cloth area. And finally, no one can climb up on top of each other, to preserve space. Everyone’s feet must be touching the piece of cloth at all times!
Problem:
Design a stack that supports push, pop, top and retrieving the minimum element in constant time
- push(x) → Push element x onto stack
- pop() → Removes the element at the top of the stack
- top() → Return the top element
- getMin/90 → Retrieve the minimum element in the stack
Given a string containing just the characters (, ), {, }, [, ] determine if the input string is valid by checking if all open brackets have a closing bracket and that they are present in the right order
Given a stream of integers and a window size, calculate the moving average of all integers in the sliding window
Given a char array representing tasks CPU need to do. It contains capital letters A to Z where different letters represent different tasks. Tasks could be done without original order. Each task could be done in one interval. For each interval, CPU could finish one task or just be idle. However, there is a non-negative cooling interval n that means between two same tasks, there must be at least n intervals that CPU are doing different tasks or just be idle. You need to return the lease number of intervals the CPU will take to finish all the given tasks.
Advanced Session
Lead - Gautam
Problem:
Given a linked list, find the middle element of the linked list
public void middle(String str) {
}
Given a linked list, an int m, and an int n, return the sum of the mth element from beginning of the list and the nth element from the end of the list
public void sumMN(int m, int n) {
}
Given a linked list, segregate the list into evens and odds in such a way that the evens are listed before odds
public void seperateOddEven() {
}
Novice Session
Lead - Sivam
Return an integer array of the values in the matrix in spiral order
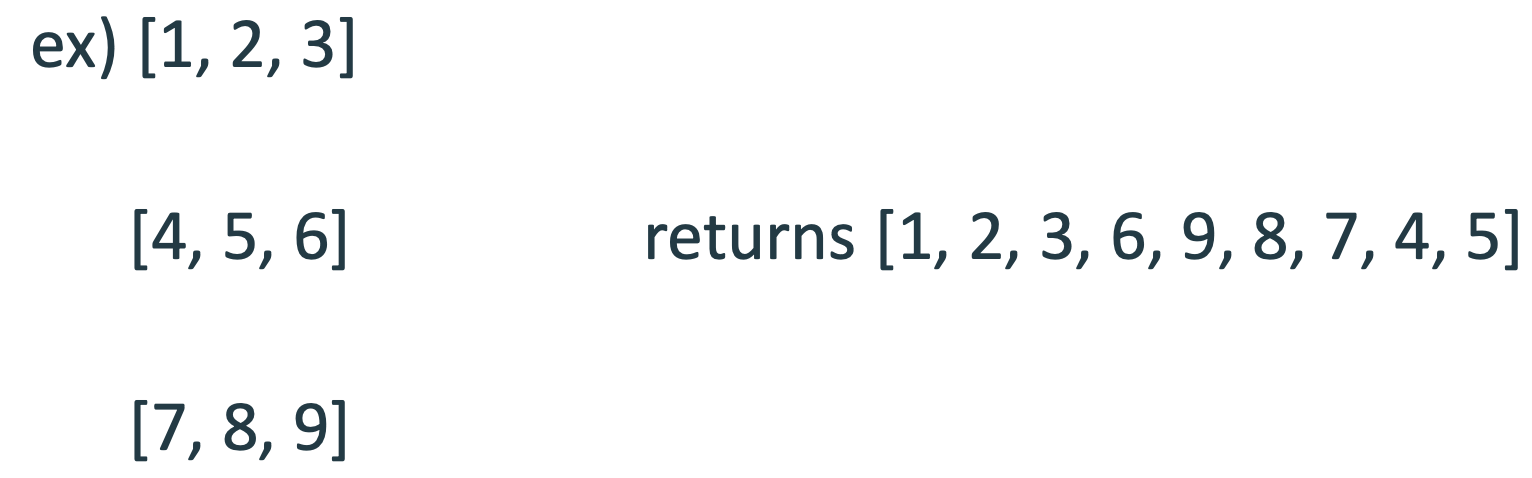
public int[] spiral(int[][] matrix) {
}
Find the longest consecutive sequence of numbers in an integer array
public int longestConsecutive(int[] nums) {
}
If you are given a sorted array of integers, return the sorted array of the squares of all values
public int sortedSquares(int[] A) {
}
Given a 2d array of characters, check if the given word exists
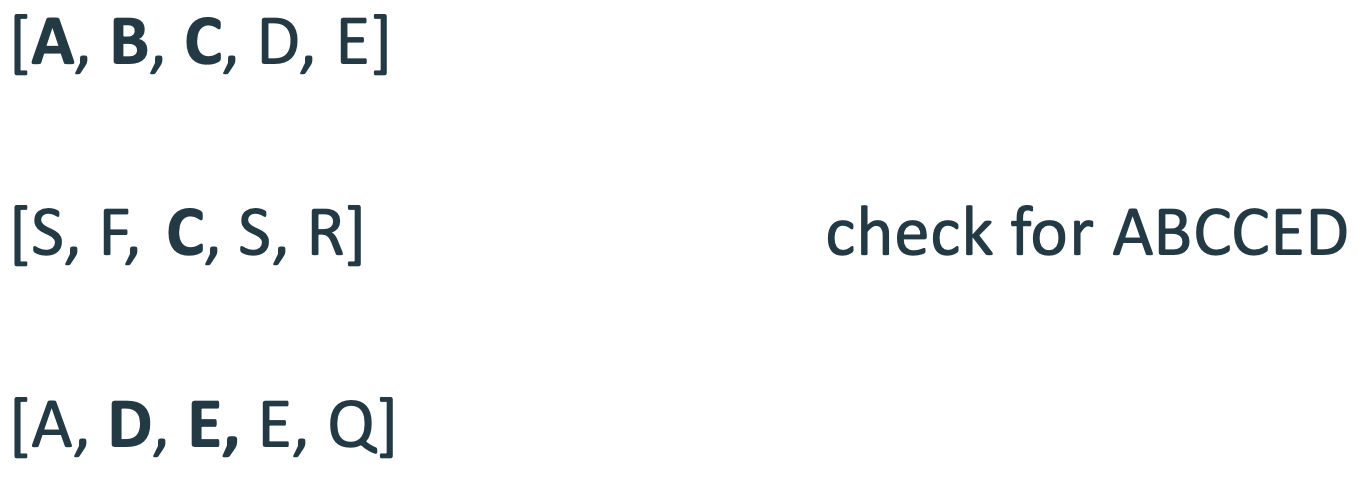
public boolean wordSearch(char[][] board, String word) {
}
Novice Session
Lead - Atharv
Problem:
Write a function to print the number of occurrences of a character in a string
public void listCountsOfCharacters(String str) {
}
Write a function that rotates an array k times to the right
public int[] rotated(int[] array, int k) {
}
Given two 2-D arrays, write a function that multiplies them
public int[][] multiplyMatrix(int[][] a, int[][] b) {
}
Novice Session
Lead - Harsha
Problem:
Write a function to compute the sum of all prime numbers less than equal to a given integer N
public int getPrimeSum (int n) { {
}
Solution: O(√N)
Write a function that returns if two strings are anagrams
public boolean isAnagram (String a, String b) {
}
Solution: O(N)
Solution: O(Nlog(N))
Suppose you had N identical balls. One of them is slightly heavier and you are given a balance scale. Write a function to return the fewest number of times you have to use the scale to find the heavier ball
public int getMinUses (int n) {
}